Note
Click here to download the full example code
Field lines with Matplotlib
Visualising traced field lines with Matplotlib.
First, load the required modules.
import astropy.units as u
import matplotlib.pyplot as plt
import numpy as np
from psipy.data import sample_data
from psipy.model import MASOutput
from psipy.tracing import FortranTracer
Load a set of MAS output files.
To trace field lines, start by creating a tracer. Then we create a set of seed points at which the field lines are drawn from.
tracer = FortranTracer()
nseeds = 5
# Radius
r = np.ones(nseeds**2) * 40 * u.R_sun
# Some points near the equatorial plane
lat = np.linspace(-10, 10, nseeds**2, endpoint=False) * u.deg
# Choose random longitudes
lon = np.random.rand(nseeds**2) * 360 * u.deg
# Do the tracing!
flines = tracer.trace(model, r=r, lat=lat, lon=lon)
flines is a list, with each item containing a field line object
print(flines[0])
FieldLine(r=<Quantity [235.01787355, 233.53256208, 232.0472505 , 230.5619389 ,
229.07662733, 227.59131582, 226.10600438, 224.620693 ,
223.13538167, 221.65007033, 220.16475896, 218.67944755,
217.19413611, 215.7088247 , 214.22351337, 212.73820213,
211.25289095, 209.76757982, 208.28226868, 206.79695751,
205.3116463 , 203.82633505, 202.34102383, 200.85571269,
199.37040165, 197.88509067, 196.39977972, 194.91446876,
193.42915775, 191.94384669, 190.4585356 , 188.97322454,
187.48791356, 186.00260269, 184.51729189, 183.03198113,
181.54667036, 180.06135955, 178.57604868, 177.09073779,
175.60542692, 174.12011615, 172.63480549, 171.14949491,
169.66418437, 168.17887383, 166.69356325, 165.20825262,
163.72294197, 162.23763136, 160.75232084, 159.26701042,
157.78170008, 156.29638978, 154.81107947, 153.32576912,
151.84045872, 150.35514831, 148.86983793, 147.38452763,
145.89921744, 144.41390733, 142.92859727, 141.44328721,
139.95797712, 138.47266701, 136.98735688, 135.50204678,
134.01673678, 132.53142689, 131.04611709, 129.56080736,
128.07549765, 126.59018794, 125.1048782 , 123.61956843,
122.13425868, 120.648949 , 119.16363943, 117.67832995,
116.19302053, 114.70771114, 113.22240176, 111.73709234,
110.2517829 , 108.76647348, 107.28116414, 105.79585492,
104.31054581, 102.8252368 , 101.33992787, 99.85461894,
98.36930997, 96.88400095, 95.39869194, 93.91338299,
92.42807415, 90.94276545, 89.4574569 , 87.97214845,
86.48684 , 85.0015315 , 83.51622292, 82.03091433,
80.54560579, 79.06029739, 77.5749892 , 76.08968125,
74.60437344, 73.11906562, 71.63375771, 70.14844969,
68.66314161, 67.17783357, 65.69252571, 64.2072182 ,
62.72191103, 61.23660402, 59.75129698, 58.26598979,
56.78068239, 55.29537483, 53.81006725, 52.32476003,
50.83945329, 49.35414697, 47.86884087, 46.38353471,
44.89822826, 43.41292143, 41.92761434, 40.44230729,
38.95700043, 37.4716938 , 35.98638724, 34.50108049,
33.01577335, 31.53046659, 30.04515629, 30.33970445,
30.99884151, 30.50381613, 30.00880618, 30.00890628,
30.00912741, 30.01044958, 31.00236984, 30.51998283,
30.52016392, 30.02611635, 30.0262427 , 31.01544684,
30.52040332, 30.02537791, 31.01561054, 30.52059804,
30.02561237, 30.02567446, 31.01568155, 30.52064752,
30.02562801, 31.01586093, 30.52086595, 30.02589606,
30.02594953, 30.02585282, 31.01608364, 30.52126568,
30.02633902, 31.01649943, 30.52146695, 30.02644991,
31.01666349, 30.52163611, 30.02662728, 31.01685809,
30.52187618, 30.52195455, 30.52181658, 30.52209627,
30.02722741, 31.01745519, 30.52252773, 30.52261766,
30.028035 , 31.01817375, 30.52313523, 30.02811058,
31.01833736, 30.52331427, 30.02831074, 31.01851319,
30.52347991, 30.02846198, 31.01867851, 30.52364815,
30.02863436, 31.01886359, 30.52386429, 30.02888129,
30.0289343 , 31.01916249, 30.52439699, 30.02945695,
31.01968359, 30.52472454, 30.52478802, 30.03001241,
31.02023784, 30.52529859, 30.52536742, 30.52546864,
30.03156478, 31.02178959, 30.52686527, 30.03190107,
30.0319538 , 30.03202922, 31.0222646 , 30.52828665,
30.52830686, 30.52856037, 30.03365829, 30.03369216,
30.03361052, 30.03357541, 31.02379823, 30.52892089,
30.52897146, 30.52882425, 30.03391446, 30.03395408,
31.02417604, 30.52938995, 30.5294296 , 30.52948434,
30.52955467, 30.52964093, 30.52990487, 30.04119298,
30.04120207, 30.04123952, 31.03145896, 30.53695926,
30.53699182, 30.53703163, 30.04262405, 30.04264605,
30.04268009, 30.04272021, 31.03293971, 30.53916293,
30.53919641, 30.53923436, 30.04490498, 30.04492838,
30.0449627 , 31.03518081, 30.54071804, 30.54075068,
30.54078899, 30.04639169, 30.04642369, 30.04646064,
30.04650497, 31.03672536, 30.54322721, 30.54325011,
30.54328937, 30.54333448, 30.5433869 , 30.54346176,
30.54382896, 30.05694773, 30.0569872 , 30.05703006,
30.05707836, 30.05713191, 30.05722374, 30.05761544,
30.0605351 , 31.05162269, 30.59109382, 30.09618321,
30.09622671, 30.09611732, 31.08634101, 30.5914739 ,
30.59152281, 30.59157054, 30.59037711, 30.59042669,
30.5900009 , 30.590051 , 30.09557071, 31.08579694,
30.59087263, 30.59092447, 30.59072756, 30.09586151,
30.09591368, 31.08613651, 30.59151829, 30.09659494,
31.08682258, 30.59186846, 30.09691019, 31.08713824,
30.59217313, 30.59222118, 30.09743377, 31.08766084,
30.59272761, 30.09777586, 31.08800389, 30.59304213,
30.09808174, 30.09813307, 30.09818354, 30.09737404,
31.08746357, 30.59242496, 30.09740243, 31.08762829,
30.59260407, 30.09760117, 31.08783104, 30.5928447 ,
30.09787606, 30.09792838, 30.09797965, 31.08820092,
32.5735049 , 34.05881115, 35.54412018, 37.02943265,
38.5147485 , 40. , 39.01144069, 37.52616098,
36.04088159, 34.5556024 , 33.07032363, 31.5850459 ,
30.09976215] solRad>, lat=<Quantity [-0.12968333, -0.12970333, -0.12972167, -0.12974095, -0.12976338,
-0.1297894 , -0.12981752, -0.12984557, -0.12987191, -0.12989559,
-0.12991702, -0.12993833, -0.12996248, -0.12999004, -0.13002023,
-0.13005108, -0.13008051, -0.13010689, -0.13012974, -0.13015047,
-0.13017206, -0.13019738, -0.13022612, -0.13025669, -0.13028706,
-0.13031503, -0.1303392 , -0.13035993, -0.13037965, -0.13040172,
-0.13042829, -0.13045763, -0.13048772, -0.13051624, -0.1305412 ,
-0.13056213, -0.13058052, -0.13059937, -0.13062158, -0.13064867,
-0.1306778 , -0.13070629, -0.13073154, -0.13075215, -0.13076848,
-0.13078251, -0.130797 , -0.13081432, -0.13083537, -0.13085698,
-0.13087695, -0.13089369, -0.13090674, -0.13091658, -0.1309245 ,
-0.1309325 , -0.13094296, -0.1309559 , -0.13096867, -0.13098029,
-0.13098996, -0.13099679, -0.13100028, -0.13100116, -0.13100154,
-0.13100373, -0.13100595, -0.13100723, -0.13100758, -0.13100605,
-0.13100136, -0.13099297, -0.13098149, -0.13096858, -0.13095561,
-0.1309414 , -0.13092713, -0.13091282, -0.13089737, -0.13087928,
-0.13085738, -0.13083175, -0.13080407, -0.13077226, -0.13073773,
-0.13070349, -0.13067058, -0.13063747, -0.1306016 , -0.13056158,
-0.13051856, -0.13047042, -0.1304153 , -0.13035728, -0.13029951,
-0.13024296, -0.13018662, -0.13012948, -0.13007263, -0.13001459,
-0.12994861, -0.1298752 , -0.12979534, -0.12971059, -0.12962398,
-0.12953909, -0.12945998, -0.12938998, -0.12932334, -0.12925413,
-0.12917714, -0.12908914, -0.12898969, -0.12888266, -0.12877823,
-0.12869718, -0.12864606, -0.12861342, -0.12858389, -0.12854171,
-0.1284759 , -0.12838908, -0.1283021 , -0.12825873, -0.12826814,
-0.1283184 , -0.12838771, -0.1284487 , -0.1284891 , -0.12852155,
-0.12857033, -0.12865047, -0.12875903, -0.12888362, -0.1289996 ,
-0.12908697, -0.12914276, -0.12916584, -0.12916589, -0.1240052 ,
-0.12277051, -0.12303445, -0.12329503, -0.12389158, -0.12491279,
-0.12662728, -0.12675235, -0.12625173, -0.12724148, -0.12739328,
-0.12822235, -0.12831562, -0.12861829, -0.12890506, -0.12904248,
-0.12934082, -0.12962938, -0.13031758, -0.13044547, -0.13074068,
-0.13102567, -0.13117211, -0.13145973, -0.13173426, -0.13240812,
-0.13326077, -0.13344221, -0.13367021, -0.13392324, -0.13403192,
-0.13430898, -0.1345762 , -0.13468872, -0.13496204, -0.13522487,
-0.13536706, -0.13562844, -0.13630045, -0.13686096, -0.137283 ,
-0.13750198, -0.13764467, -0.13787689, -0.13857163, -0.13873384,
-0.13882878, -0.13908002, -0.1393206 , -0.13943185, -0.13967751,
-0.13991103, -0.14000225, -0.14024518, -0.14047761, -0.14057519,
-0.14081421, -0.14104221, -0.14116307, -0.14139235, -0.14160969,
-0.14212367, -0.14228441, -0.14244725, -0.14264682, -0.14276781,
-0.1429745 , -0.14354768, -0.14370556, -0.14382295, -0.14401643,
-0.14459353, -0.14541495, -0.14545433, -0.14556828, -0.14574448,
-0.1459247 , -0.14639295, -0.147061 , -0.14729407, -0.14731389,
-0.14783435, -0.14859019, -0.1487321 , -0.14915142, -0.14963201,
-0.15008135, -0.15018383, -0.15030983, -0.15077582, -0.15155568,
-0.15167222, -0.1520499 , -0.15215702, -0.15224661, -0.15267377,
-0.15321954, -0.15395699, -0.15487517, -0.15614705, -0.1558108 ,
-0.15611708, -0.15650098, -0.15661386, -0.15662163, -0.15696649,
-0.15740767, -0.1573913 , -0.15766617, -0.15802965, -0.15853405,
-0.15868483, -0.1586083 , -0.15892241, -0.15935694, -0.15931625,
-0.15957491, -0.1599278 , -0.16002688, -0.16001161, -0.16031687,
-0.16072773, -0.16068737, -0.1609588 , -0.16134274, -0.16187125,
-0.16202699, -0.16192703, -0.16223189, -0.16261399, -0.16313738,
-0.16379293, -0.16460031, -0.16608601, -0.16557065, -0.16584784,
-0.16625169, -0.16678534, -0.16743047, -0.16826245, -0.1698351 ,
-0.17200819, -0.17228342, -0.17122194, -0.17120419, -0.17142774,
-0.17162924, -0.17164955, -0.17166619, -0.17192307, -0.17229734,
-0.17299963, -0.17328654, -0.17364182, -0.17392783, -0.1738253 ,
-0.17382602, -0.17384333, -0.17407677, -0.17430758, -0.17426648,
-0.174482 , -0.17452176, -0.17449185, -0.17446247, -0.17445314,
-0.17447228, -0.17445043, -0.17443811, -0.17445885, -0.17466305,
-0.1746055 , -0.17460071, -0.174616 , -0.17459225, -0.1745802 ,
-0.17459989, -0.174578 , -0.17477276, -0.17507386, -0.17551207,
-0.17544138, -0.17546172, -0.17545237, -0.17541305, -0.17542908,
-0.17541209, -0.17538802, -0.17540616, -0.17538209, -0.17556713,
-0.17585697, -0.17592258, -0.17581958, -0.17575877, -0.17570807,
-0.17555456, -0.17522675, -0.17453293, -0.17273518, -0.1725799 ,
-0.17246801, -0.17238233, -0.17231193, -0.17226346, -0.17224354] rad>, lon=<Quantity [4.99459915, 4.99993125, 5.00523583, 5.01053265, 5.01583664,
5.02115769, 5.02649934, 5.03185929, 5.03722957, 5.04260008,
5.04796201, 5.05331154, 5.05865223, 5.0640026 , 5.06937402,
5.07476977, 5.08018557, 5.08561173, 5.09103692, 5.09645255,
5.10185585, 5.10725095, 5.11265442, 5.11807904, 5.12352809,
5.12899639, 5.13447303, 5.13994609, 5.14540718, 5.15085394,
5.1562909 , 5.16173664, 5.1672056 , 5.17270181, 5.17821972,
5.18374752, 5.18927241, 5.19478549, 5.20028438, 5.20577416,
5.21127407, 5.21679862, 5.22235194, 5.22792816, 5.23351514,
5.2391002 , 5.24467512, 5.25023846, 5.25579591, 5.26136295,
5.26695332, 5.27257128, 5.278211 , 5.28386039, 5.28950716,
5.29514373, 5.30076901, 5.30638859, 5.31201694, 5.31766752,
5.32334542, 5.32904589, 5.33475786, 5.34047 , 5.34617547,
5.35187328, 5.3575674 , 5.36327117, 5.36899786, 5.37475361,
5.38053516, 5.38633258, 5.39213533, 5.39793685, 5.40373251,
5.40951997, 5.41531246, 5.42112355, 5.42696062, 5.43282177,
5.43869874, 5.44458281, 5.45046816, 5.4563452 , 5.46221616,
5.46809316, 5.47399012, 5.4799162 , 5.48587189, 5.49185146,
5.49784835, 5.50384837, 5.5098363 , 5.51581388, 5.52179196,
5.5277856 , 5.53380747, 5.53986352, 5.54595379, 5.5520703 ,
5.55818822, 5.56429129, 5.57037766, 5.57645833, 5.58255273,
5.58868161, 5.59486049, 5.60109657, 5.60736647, 5.61363491,
5.6198807 , 5.62609972, 5.6323044 , 5.63851992, 5.64477746,
5.65111731, 5.6575373 , 5.66399403, 5.67044523, 5.6768599 ,
5.68322568, 5.68955329, 5.6958789 , 5.70228595, 5.7088057 ,
5.71541948, 5.72208095, 5.72873025, 5.73531504, 5.74181301,
5.74825224, 5.754698 , 5.76119012, 5.76773276, 5.77428988,
5.78080732, 5.78723372, 5.79374743, 5.79936363, 5.37256789,
5.60571412, 5.604344 , 5.60258194, 5.59253602, 5.57160309,
5.52416894, 5.49077946, 5.59338044, 5.57447493, 5.59744985,
5.58156123, 5.60476816, 5.60540167, 5.60421922, 5.60053691,
5.60005243, 5.60092414, 5.59451288, 5.60406862, 5.6037773 ,
5.60308326, 5.59793333, 5.59851644, 5.60031561, 5.59453221,
5.5957152 , 5.58802454, 5.59557801, 5.59940168, 5.6029612 ,
5.60266421, 5.60237708, 5.60183735, 5.60145641, 5.60163998,
5.59597781, 5.5975879 , 5.58901587, 5.59230507, 5.59244884,
5.59869011, 5.59208565, 5.59626326, 5.58365687, 5.59778146,
5.60248798, 5.60282068, 5.60262919, 5.59984596, 5.60000108,
5.60091525, 5.6014616 , 5.60157185, 5.60181475, 5.60079545,
5.600926 , 5.60143841, 5.59648592, 5.59790901, 5.60001654,
5.59277002, 5.58505735, 5.59501846, 5.59912392, 5.59309609,
5.59654932, 5.58832914, 5.59802657, 5.59176919, 5.59616892,
5.58589305, 5.57038761, 5.59711931, 5.59071682, 5.59591605,
5.59937355, 5.59080567, 5.57736386, 5.56810493, 5.59400557,
5.59017849, 5.59228998, 5.59854551, 5.59316497, 5.59489589,
5.59462069, 5.58794084, 5.59542707, 5.58707401, 5.5922259 ,
5.59858192, 5.5915296 , 5.58440419, 5.5949868 , 5.58857639,
5.57662691, 5.56258646, 5.54712406, 5.5235198 , 5.59668802,
5.59377959, 5.58404342, 5.57639491, 5.59445281, 5.58825163,
5.57760742, 5.59740429, 5.59282834, 5.58325932, 5.57208809,
5.56380917, 5.59402283, 5.58651729, 5.57582171, 5.5971724 ,
5.59231441, 5.58267478, 5.57508535, 5.59400325, 5.58742322,
5.57686171, 5.59684646, 5.5898191 , 5.57955525, 5.56785311,
5.55936339, 5.59288471, 5.58840035, 5.57798557, 5.56596866,
5.55291432, 5.5381357 , 5.51115157, 5.59470674, 5.58573735,
5.57458507, 5.56238124, 5.54947884, 5.53396778, 5.50660354,
5.43811125, 5.41409345, 5.5888401 , 5.59468972, 5.58730108,
5.59049859, 5.58369392, 5.59087255, 5.58114836, 5.56922409,
5.58755371, 5.57646652, 5.58694099, 5.57568802, 5.59236792,
5.58592153, 5.59064897, 5.58078161, 5.58644585, 5.59318529,
5.58320444, 5.57542235, 5.58895829, 5.5936002 , 5.58750765,
5.59089982, 5.59412206, 5.58822211, 5.59114564, 5.58352855,
5.59265592, 5.58626821, 5.59048176, 5.5939447 , 5.58797533,
5.59101139, 5.59410808, 5.58549955, 5.5738207 , 5.58985772,
5.59662046, 5.59700358, 5.59672536, 5.59433731, 5.59434969,
5.59517203, 5.58974621, 5.59147225, 5.59398251, 5.58544599,
5.57370921, 5.56537471, 5.55825812, 5.55163156, 5.54566163,
5.54062598, 5.53672987, 5.54407744, 5.5963267 , 5.60740715,
5.61852982, 5.62968023, 5.64088664, 5.65222972, 5.662741 ] rad>)
To easily visualise the result, here we use Matplotlib. Note that Matplotlib is not a 3D renderer, so has several drawbacks (including performance).
fig = plt.figure()
ax = fig.add_subplot(projection="3d")
br = model["br"]
for fline in flines:
# Set color with polarity on the inner boundary
color = (
br.sample_at_coords(
np.mod(fline.lon[0], 2 * np.pi * u.rad),
fline.lat[0],
fline.r[0],
)
> 0
)
color = {0: "red", 1: "blue"}[int(color)]
ax.plot(fline.xyz[:, 0], fline.xyz[:, 1], fline.xyz[:, 2], color=color, linewidth=2)
lim = 60
ax.set_xlim(-lim, lim)
ax.set_ylim(-lim, lim)
ax.set_zlim(-lim, lim)
plt.show()
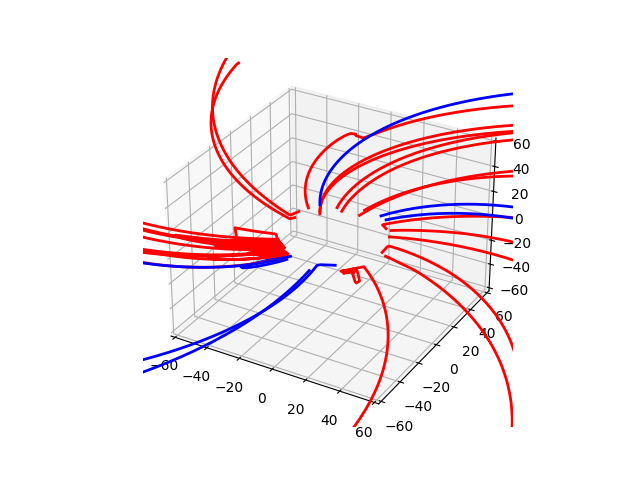
Total running time of the script: ( 0 minutes 0.988 seconds)